Perhaps you misunderstood setTimeout
setTimeout
is used for executing some logic after a certain time. But exactly how long do we have to wait for that logic to be executed?
How does JS engine execute our code?
JS engine executes code in single thread (main thread). It has something called Call stack. Code will be executed in a last-in, first-out (LIFO) order in which it is called. It means that, if some logic is being executed, other logic will have to wait.
console.log(1);
console.log(2);
console.log(3);
// Output
1
2
3
Some logic that can take a long time to execute like I/O, network, ... will be executed in other threads to prevent blocking main thread. They're asynchronous tasks.
What is Event loop?
We must hear about Event loop. Event loop is the secret mechanism behind how JS code is executed. Image below describes how Event loop works in browser.
Event loop in browser is different from one in NodeJS. We will mention Event loop in browser in example below.
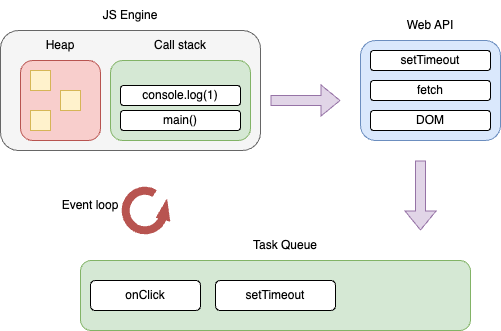
Basically, when a task is executed, it will be pushed into Call stack.
If it is synchronous task, it will be executed immediately and popped out of stack.
If it is asynchronous task like setTimeout
, setInterval
, fetch
, ... it will be assigned to browser and browser will pick other threads to execute it.
Functions like setTimeout
, setInterval
, fetch
, ... are not available in JS Engine. They are a part of JS runtime APIs.
After being executed, its callback will be pushed into Task queue. This is when Event loop does its job. Whenever Call stack is empty, it will pick the first task in Task queue into Call stack to execute.
Event loop is infinite loop which monitors the Call stack and Task queue.
The moment of the truth
As we know above, a queued task is only picked and executed only when the Call stack is empty.
So, if we call a setTimeout
function
setTimeout(cb, 100)
After 100ms, cb
will be pushed into Task queue and ready to execute in Call stack.
But if Call stack is handling task A
which takes about 1 min, cb
will have to wait for 1 min as well.
Conclusion
Hope this post help you gain a deeper insight about setTimeout
.
Reference
Thank you for reading. Welcome all your comments/feedbacks.